Frequently Asked Questions
General
Installation
Administration
How to make my website public?
To put your website on internet, you need to change host, ip and port of your AIDASite, but first:
1. Be sure your computer has a public IP address, which is static, not dynamic. Be sure also that you are not behind a firewall, otherwise you need to setup your firewall/router for port forwarding. To see if you are not behind firewall, check IP and if it is 192.168.x.x or 10.x.x.x, then you are surelly behind a firewall.
2. You need to register your hostname in some DNS server. For example, for a www.mysite.org and IP 242.34.11.1 you need to have a domain mysite.org open on some DNS server and you need to add entry:
www IN A 242.34.11.1
3. Check that above hostname is active: ping www.mysite.org
4. Setup your AIDASite (let say it is named 'mysite') with correct host/ip/port trio:
(AIDASite named: 'mysite') host: 'www.mysite.org' ip: '242.34.11.1' port: 80
5. Restart Aida, astually a Swazoo webserver:
SwazooAida stop; start.
How to open another website?
By default we have a site named 'aidademo'. Let we add another site named 'mysite':
AIDASite newNamed: 'mysite'
Now we need to set host/ip/port of the new wesite. Since default one has host 'localhost' ip '127.0.0.1' port 8888, let we just run the new site on different port 9999:
(AIDASite named: 'mysite') host: 'localhost' ip: '127.0.0.1' port: 9999.
Now restart the underlying Swazoo web server:
SwazooAida stop; start.
... and open new website: http://localhost:9999
What is a default website?
For convenience one of the websites can be set as default and then you can access it more easily:
AIDASite default
You set a site as default:
AIDASite default: 'mysitename'
Besides AIDASite you can access that way also URLResolver default, WebSecurityManager default, WebSessionManager default, WebStatistics default
How to monitor/ping my website?
Every Aida website has a reserved Url /ping.html designated to monitor your website from tools like Nagios. "Pinging" that Url won't cause those requests to be counted by Aida statistics.
Programming
How to make nice looking URLs?
By default Aida is creating Urls automatically in format like that: /object/o24552334.html. But you can direct the Url creation by adding the method #preferedUrl to your domain object, which must return a relative Url of that object. For instance, for class Person you'll implement a method like:
Person>>preferedUrl
^'/person/', self name, '.html'
Be careful, only one r in preferedUrl!
How to hide/show some web element?
Say you have a comment form on your blog and you'd like to initially hide it and show when use clicks on 'comment' link.
1. make an element with comment form:
BlogPostApp>>commentForm
e := WebElement new.
...
^e
2. In the main view of blog post add a dummy/nil link to click o it and initially hidden comment form will be shown
BlogPostApp>>viewMain
"... main blog post part... "
comment := self commentForm.
comment hide.
(self addNilLinkText: 'comment') onClickToogle: comment
self add: comment.
How to pass additional parameters in URLs?
When adding links in your code, add a parameter to it, for example, if you like to add some unique identifier (uuid) to the link:
e addLinkTo: self observee view: #byUuid parameter: 'uuid' value: someObject uuid
Then read this value from request, for this example in view method:
MyApp>>viewByUuid
...
uuid := self session lastRequest queryAt: 'uuid'
object := self observee objectWithUuid: uuid.
...
You can read such values from request in action methods or elsewhere too.
To add more that one parameter, cascade more #parameter:value calls to the link:
(e addLinkTo: self observee view: #byUuid)
parameter: 'uuid' value: someObject uuid;
parameter: 'date' value: Date today printString.
Auto conversion of input field values?
There is auto convertion support in Aida for dates, integers and fixed points to ease accessing data in domain models. But it has some restrictions. First, an expected format is fixed, for numbers 123.456,00 (comma as decimal separator) and for dates in dd.mm.yyyy format. Date format also allows a bit more flexibility, like without points: ddmm (for current year), ddmmyy.
To support additional data types or different formats you need to introduce your own accesor methods which make an appropriate convertion. For float for instance:
aDomainObject float: 12.3; float
you add aditional accessors with conversions inside:
aDomainObject floatText: '12.3'; floatText
Then you use floatText instead of float accesors to use input fields:
e addInputFiledAspect: #floatText for: self observee
Styling
What is WebStyle?
Aida separates also design from content. Design, or "styling" is put in a class WebStyle and you can change it by subclasing WebStyle and registering it in your AIDASite (see next question).
In WebStyle there are methods which contain CSS, method images, also JavaScript and more. Here is also a place for header, menus and other stuff which is common to all pages on the website. All this defines the default style of Aida website. Study class WebStyle to understand that better.
How to use my own style?
Subclass WebStyle with your own one, say MyWebStyle. Then change style of your website (let say it is named 'mysite'):
(AIDASite named: 'mysite') styleClass: 'MyWebStyle
Now you start adding or overriding your own CSS, method images, also your own header and frame navigation etc.
What is the URL of method images?
All method images have an URL composed by the convention. For example the method image #arrowBigRedGif in DefaultWebStyle (category imgs-bullets): is accessible by the relative URL:
'/img/arrowBigRedGif.gif'
We need to use relative URLs in CSS, while in app code we should use just name of the image method as argument of image adding methods (#addGif: #addPng: #addJpeg: ), for example:
e addGif: #arrowBigRedGif
How to change the banner in page header?
After Aida installation and running , the first thing desirable may be to change the Aida banner with your own one.
First prepare your own image with the same size as current one and save it as .gif. Now you also need to "save" it into so called method image, that is, a method in WebStyle or subclass, which will contain an array of bytes of that image. To do that you can use a WebStyle maintenance method #importImage:from: .
Let we override a banner image in our own WebStyle subclass, with an image aidaBanner.gif, put in directory from where the image is run:
MyWebStyle new import: 'aidaBannerGif' from: 'aidaBanner.gif'
After page refresh you should see a new banner in page header:
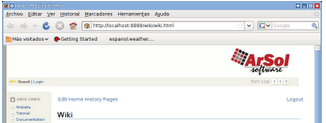
How to change favicon?
Favicon or favorites icon is that small image shown near Url in browser location field, also in tabs and bookmarks/favorites. By default Aida shows next favicon:

To change it you need to override a #favicon method in your WebStyle subclass:
MyWebStyle new importImage: 'favicon' from: 'favicon.ico'
This call will create new (or override existing) method #favicon in category imgs. Currently only .ico format is supported!
Advanced
Returning content of different types
Returning an answer in a content type other that HTML is actually pretty simple. Let me explain with example returning an XML:
1. make a new class, say XMLContent as subclass of WebElement
2. ovveride method #aidaContentType to return appropriate content type:
XMLContent>>aidaContentType
^'text/xml'
3. MyApp>>viewAsXml
| xml |
xml := '<xml>some XML content</xml>.
e := XMLContent new.
e addText: xml.
^e
4.in browser open something like:
http://localhost:8888/myobject?view=asXml
To explain a bit: if App view method return an element with content type different than default, this content type will be set in a header of response to your web request. This way we can very simply return content in any content type we like, like XML, CVS, JPG etc.